Java OOP basics
- Shakil
- Mar 1, 2018
- 5 min read
This blog will help you to achieve the basic foundation of object oriented programming in java.

Object and Class
In simplicity an object which represents the real entity of a class. And in OOP object is the instance of class. In java class has some properties, there can be methods, class variables and inner classes.
Rahim is a human. He has hand, leg, a nose, a head and so on. We can say Rahim is an instance of human class. See we all have the same body parts as Rahim has. So we are also the instances of human class. Finally sort it out,
Class is the blue print or the default architecture of an object.
Object is the instance or reference of a class.
Let’s see an example:
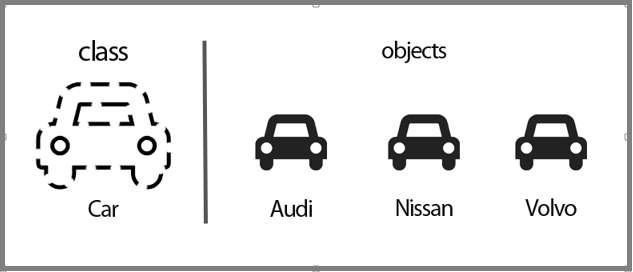
In this image we can see that car which represents as a class. Where we can see that it has a default architecture.
On the other side there are some cars from different brands. They are as Audi,Nissan,Volvo.These brands are objects. They take the default car structure from car class and build their cars.
Encapsulation
You are sending some private messages to someone and you don’t want to share this messages with someone or you don’t want that someone sees your messages. So you choose the Secret Message option from your app so that your security remains the way you want.
Encapsulation is an OOP feature which helps to hide data from unwanted uses. It is all about wrapping variables and methods in one single unit with the sole purpose of data hiding from external classes.
We ensure encapsulation in java with the help of access modifiers. Java has basically 4 access modifiers. They are as follows,
Public
Private
Protected
Default
Public:
A class, method, constructor, interface, etc. declared public can be accessed from any other class. It can be accessible from any class within the same package.
Private:
Methods, variables, and constructors that are declared private can only be accessed within the declared class itself. It ensures the highest level of security in your program.
Protected:
Variables, methods, and constructors, which are declared protected in a superclass can be accessed only by the subclasses in other package or any class within the package of the protected members' class.
Default:
Default access modifier is package-private - visible only from the same package.
Access levels of modifiers in java:
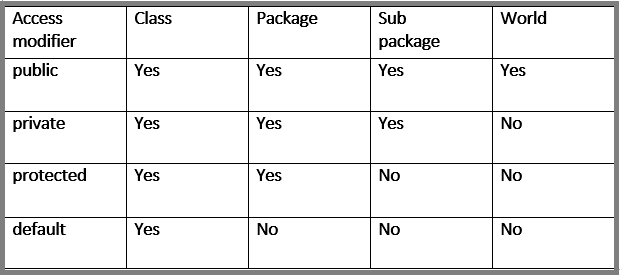
Consider an example as,
You create two classes one is teacher and another is student. Students has some features like show results, registered subjects and so on. On the other hand teacher has also some features. Now you want that student and teacher should remain separate. They should not violate their access.
So, you keep the variables of both classes as private with the help of private access modifier.


Simply you just add private access modifier before the datatype of each variable. So that is how you can ensure encapsulation in java by the help of access modifier.
Link that will help you:
http://www.wideskills.com/java-tutorial/introduction-to-java-access-modifiers
Inheritance
Inheritance is such a useful property of object oriented programming, which helps us in maximum code re usability. Inheritance enables new object to take on the property of another object. The aim of inheritance is to provide the re usability of code so that a class has to write only the unique features and rest of the common properties and functionalities can be extended from the another class.
It has two important property.
Ø Super class or parent class or base class. (The class that extends the features of another class is known as child class, sub class or derived class.)
Ø Sub class or child class or derived class. (The class whose properties and functionalities are used (inherited) by another class is known as parent class, super class or Base class.)
Why we use inheritance:
Ø We use inheritance to ensure maximum code reusability.
Ø In order to organize the class properly.
Ø Use extends keyword to inherit super class by the sub classes.
Ø Categorize the data and which helps in efficient coding.
Ø Super class contains the generalize information
Ø Sub classes contains the specialized information or their individual information. (Any sub class must have at least one specialize property.)
It just work as like , think of a child .The baby child can inherit certain traits from his parents and as well, due to certain environmental changes develop new traits or lose traits he acquired from his parents.
Considering the previous example, we had two classes one is teacher and another one is student.
Student class has the name,cgpa,fathers name and mothers name also teacher class has name, teacher initial and department.
So let’s break down the common information between the two classes.
Ø Teacher has name and department,
Ø Student also has name and department.
So we can keep this two which is common information into a super class as follows.
Child class 1:
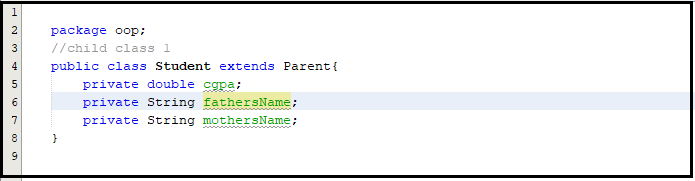
Child class 2:
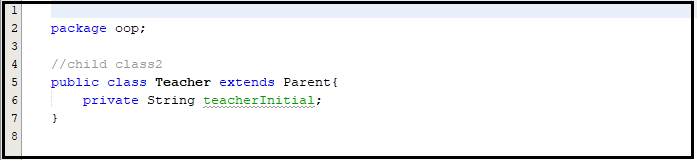
And the two child classes will extends this Parent class by the keyword extends.

Abstraction
Abstraction is one of the key concepts of object-oriented programming (OOP) languages. Its main goal is to handle complexity by hiding unnecessary details from the user.
Abstractions main job is to hide the unnecessary data from other class. In inheritance whenever you extends a class you can simply get that class data. But what happens when you extends a class and creates an object of it, which is wrong, and abstraction ensures or prevent to create the object of a class.
Abstraction has two parts:
Ø Abstract class.
Ø Abstract method.
What is Abstract class in Java? A class that is declared as abstract is known as abstract class. Syntax: abstractclass <class-name>{}
An abstract class is something which is incomplete and you cannot create instance of abstract class. If you want to use it you need to make it complete or concrete by extending it.
A class is called concrete if it does not contain any abstract method and implements all abstract method inherited from abstract class or interface it has implemented or extended.
What is Abstract method in Java?
A method that is declare as abstract and does not have implementation is known as abstract method. If you define abstract method than class must be abstract. Syntax:
abstract return_type method_name ();
An abstract method in Java doesn't have body, it’s just a declaration. In order to use abstract method you need to override that method in Subclass.
So continuing the previous example, the parent class now is our abstract class as we defined in the following picture.
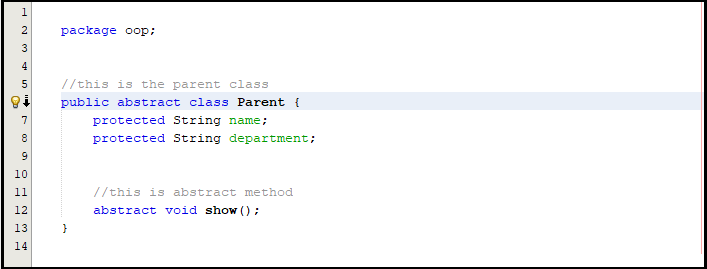
Also we declare an abstract method within our class. And as the rules we know that if we do not override this method into the child classes we will be getting error.
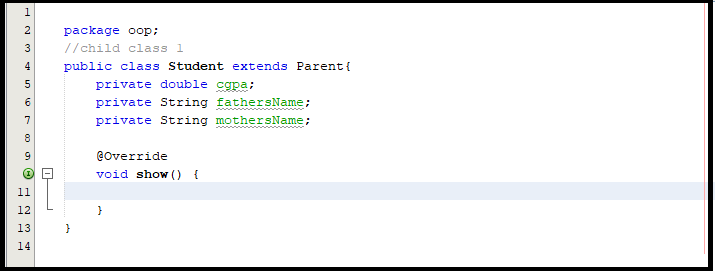
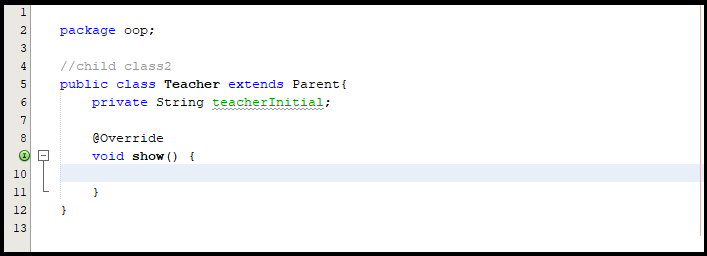
Polymorphism
This post provides the theoretical explanation of polymorphism with real-life examples. For detailed explanation on this topic with java programs refer polymorphism in java and runtime and compile time polymorphism.
· Polymorphism means to process objects differently based on their data type.
· In other words it means, one method with multiple implementation, for a certain class of action. And which implementation to be used is decided at runtime depending upon the situation (i.e., data type of the object)
· This can be implemented by designing a generic interface, which provides generic methods for a certain class of action and there can be multiple classes, which provides the implementation of these generic methods.
Polymorphism could be static and dynamic both. Method overloading is static polymorphism while, method overriding is dynamic polymorphism.
· Overloading in simple words means more than one method having the same method name that behaves differently based on the arguments passed while calling the method. This called static because, which method to be invoked is decided at the time of compilation
· Overriding means a derived class is implementing a method of its super class. The call to overridden method is resolved at runtime, thus called runtime polymorphism
May be we all know what is constructor.
We can see that here we have two methods but the method name is same. And in java there is some circumstances based on that method overloading happened.
And they are:
No of parameters
Sequence of parameters
Positions of the parameters.
Constructor has two types (one is default which is without parameters and another one is with parameters.)
For the previous example the overloading will be look like as,
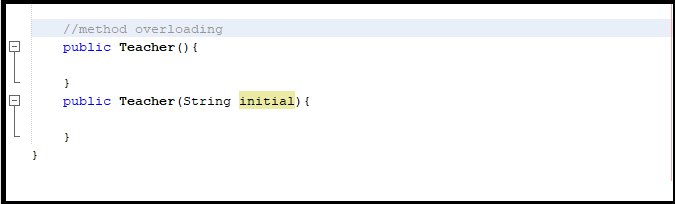
So that is it.Hope this blog will help you to know those basic things about the object oriented programming in java.
Happy Coding
Comments